Collision (AbK)
Farben
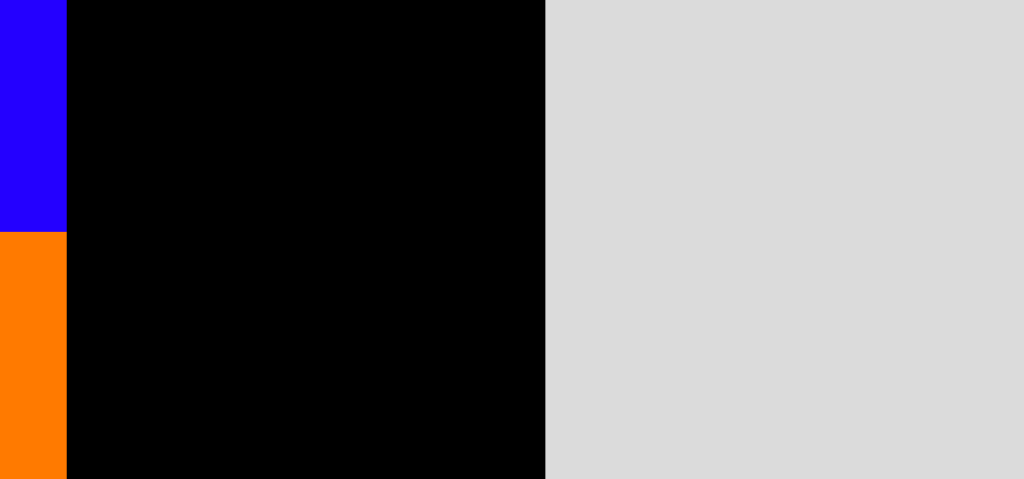
color orange = #FF7A00;
color blue = #2400FF;
color black = #000000;
color white = #DBDBDB;
Fonts
– https://fonts.google.com/specimen/Poppins
Images
Links
Step 02
color orange = #FF7A00;
color blue = #2400FF;
color black = #000000;
color white = #DBDBDB;
PImage img;
PImage[] images;
float[] yPos;
void setup() {
size(1080 / 2, 1920 / 2);
images = new PImage[5];
yPos = new float[5];
pixelDensity(2);
images[0] = loadImage("1.png");
images[0].resize(500, 0);
yPos[0] = 100;
images[1] = loadImage("2.png");
images[1].resize(500, 0);
yPos[1] = 100;
images[2] = loadImage("3.png");
images[2].resize(500, 0);
yPos[3] = 100;
images[3] = loadImage("4.png");
images[3].resize(500, 0);
yPos[3] = 100;
images[4] = loadImage("5.png");
images[4].resize(500, 0);
yPos[4] = 100;
}
void draw() {
background(white);
imageMode(CENTER);
for (int i = 0; i < images.length; i++) {
float wave = tan(radians(frameCount + i * 5)) * 50;
image(images[i], width/2 + wave, yPos[i]);
}
//saveFrame("out/####.jpg");
}
Rotation 1
color orange = #FF7A00;
color blue = #2400FF;
color black = #000000;
color white = #DBDBDB;
PImage img;
PImage[] images;
float[] yPos;
void setup() {
size(1080 / 2, 1920 / 2);
images = new PImage[5];
yPos = new float[5];
pixelDensity(2);
for (int i = 0; i < images.length; i++) {
int s;
images[i] = loadImage(i + ".png");
s = int(random(0, width));
images[i].resize(s, 0);
yPos[i] = random(0, height);
}
}
void draw() {
background(white);
imageMode(CENTER);
for (int i = 0; i < images.length; i++) {
float wave = tan(radians(frameCount + i * 5)) * 50;
push();
translate(width/2 + wave, yPos[i]);
rotate(radians(wave));
image(images[i],0,0);
pop();
}
//saveFrame("out/####.jpg");
}
Sequenzielles Laden von Bildern
void setup() {
size(1080,1080);
}
void draw() {
background(0);
PImage img = loadImage(frameCount + ".png");
// PImage img = loadImage("../../images/" + frameCount + ".png");
image(img,0,0,width,height);
}
utilities.pde
// trcc utilities version 1.2
import com.hamoid.*;
VideoExport videoExport;
/*
------------------------------------------
Basics
------------------------------------------
*/
// Sketchname
final String sketchname = getClass().getName();
// Timestamp
String timestamp() {
int y = year(); // 2003, 2004, 2005, etc.
int m = month(); // Values from 1 - 12
int d = day(); // Values from 1 - 31
int h = hour(); // 2003, 2004, 2005, etc.
int mi = minute(); // 2003, 2004, 2005, etc.
int sec = second();
int mill = millis();
String val =
"_" +
String.valueOf(y) +
String.valueOf(m) +
String.valueOf(d) + "_" +
String.valueOf(h) + "_" +
String.valueOf(mi) + "_" +
String.valueOf(sec) + "_" +
String.valueOf(mill);
return val;
}
/*
------------------------------------------
Video Export
------------------------------------------
*/
// Simple
void rec(int rate, int dur) {
if (frameCount == 1) {
videoExport = new VideoExport(this, "../"+sketchname + ".mp4");
videoExport.setFrameRate(rate);
videoExport.startMovie();
}
videoExport.saveFrame();
if (frameCount == dur) {
exit();
}
println(frameCount);
}
// Iterative
void rec(int rate, int dur, boolean timestmp) {
if (frameCount == 1) {
if (timestmp) {
videoExport = new VideoExport(this, "../"+sketchname + timestamp() + ".mp4");
} else {
videoExport = new VideoExport(this, "../"+sketchname + ".mp4");
}
videoExport.setFrameRate(rate);
videoExport.startMovie();
}
videoExport.saveFrame();
if (frameCount == dur) {
exit();
}
println(frameCount);
}
/*
------------------------------------------
Generate Thumbnail
------------------------------------------
*/
void thumb(int count) {
if (frameCount == count) {
saveFrame("../" + sketchname + ".png");
}
}
/*
------------------------------------------
Math utilities
------------------------------------------
*/
// Counter
int counter = 0;
void count(int modulo, int min, int max, boolean log) {
if (frameCount % modulo == 0) {
if (counter >= max) {
counter = min;
} else {
counter++;
}
}
if (log == true) {
println(counter);
}
}
// Function: sum of an array
float arrSum(float[] arr) {
float sum = 0;
for (int i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}