Processing Snippets
Default Camera
float eyeX = width/2.0;
float eyeY = height/2.0;
float eyeZ =(height/2.0) / tan(PI*30.0 / 180.0);
float centerX = width/2.0;
float centerY = height/2.0;
float centerZ = 0;
float upX = 0;
float upY = 1;
float upZ = 0;
camera(eyeX, eyeY, eyeZ, centerX, centerY, centerZ, upX, upY, upZ);
Video Export Setup
import com.hamoid.*;
VideoExport videoExport;
void setup(){
videoExport = new VideoExport(this, "export.mp4");
videoExport.setFrameRate(30);
videoExport.startMovie();
}
void draw(){
videoExport.saveFrame();
}
Gif-Export
// Example:
// gif(50,1,500);
import gifAnimation.*;
int gifframecount;
String filename;
String timestamp;
GifMaker gifExport;
void gif(int frames, int gifFrameModulo, int _delay) {
filename = timestamp = year() + nf(month(),2) + nf(day(),2) + "-" + nf(hour(),2) + nf(minute(),2) + nf(second(),2);
if (frameCount == 1) {
int delay;
delay = _delay;
println("gifAnimation " + Gif.version());
gifExport = new GifMaker(this, "a.gif");
gifExport.setRepeat(0);
gifExport.setDelay(delay);
}
if (frameCount % gifFrameModulo == 0) {
gifExport.addFrame();
gifframecount++;
}
if (frameCount / gifFrameModulo == frames) {
gifExport.finish();
println("gif saved");
exit();
}
}
copy()
int sx = x;
int sy = y;
int sw = tileW;
int sh = tileH;
int dx = sx;
int dy = sy;
int dw = tileW;
int dh = tileH;
comp.copy(pg, sx, sy, sw, sh, dx, dy, dw, dh);
Video-Export
import com.hamoid.*;
VideoExport videoExport;
void videoExport(int frameR, int duration) {
if (frameCount == 1) {
videoExport = new VideoExport(this, "export.mp4");
videoExport.setFrameRate(frameR);
videoExport.startMovie();
}
if (frameCount < duration){
videoExport.saveFrame();
} else {
exit();
}
}
Textured Box
void tcube(PImage tex, PGraphics surface) {
surface.beginShape(QUADS);
surface.texture(tex);
// Given one texture and six faces, we can easily set up the uv coordinates
// such that four of the faces tile "perfectly" along either u or v, but the other
// two faces cannot be so aligned. This code tiles "along" u, "around" the X/Z faces
// and fudges the Y faces - the Y faces are arbitrarily aligned such that a
// rotation along the X axis will put the "top" of either texture at the "top"
// of the screen, but is not otherwised aligned with the X/Z faces. (This
// just affects what type of symmetry is required if you need seamless
// tiling all the way around the cube)
// +Z "front" face
surface.vertex(-1, -1, 1, 0, 0);
surface.vertex( 1, -1, 1, 1, 0);
surface.vertex( 1, 1, 1, 1, 1);
surface.vertex(-1, 1, 1, 0, 1);
// -Z "back" face
surface.vertex( 1, -1, -1, 0, 0);
surface.vertex(-1, -1, -1, 1, 0);
surface.vertex(-1, 1, -1, 1, 1);
surface.vertex( 1, 1, -1, 0, 1);
// +Y "bottom" face
surface.vertex(-1, 1, 1, 0, 0);
surface.vertex( 1, 1, 1, 1, 0);
surface.vertex( 1, 1, -1, 1, 1);
surface.vertex(-1, 1, -1, 0, 1);
// -Y "top" face
surface.vertex(-1, -1, -1, 0, 0);
surface.vertex( 1, -1, -1, 1, 0);
surface.vertex( 1, -1, 1, 1, 1);
surface.vertex(-1, -1, 1, 0, 1);
// +X "right" face
surface.vertex( 1, -1, 1, 0, 0);
surface.vertex( 1, -1, -1, 1, 0);
surface.vertex( 1, 1, -1, 1, 1);
surface.vertex( 1, 1, 1, 0, 1);
// -X "left" face
surface.vertex(-1, -1, -1, 0, 0);
surface.vertex(-1, -1, 1, 1, 0);
surface.vertex(-1, 1, 1, 1, 1);
surface.vertex(-1, 1, -1, 0, 1);
surface.endShape();
}
Load all images from a specific folder and put them into a new ArrayList as a PImage
import java.io.File;
String [] imageFileNames;
ArrayList images;
void setup() {
java.io.File folder = new java.io.File(dataPath("images"));
imageFileNames = folder.list();
images = new ArrayList<PImage>();
println("loading...");
// Load the image-files and push thems to imagess-arrayList
for (int i = 0; i < imageFileNames.length; i++) {
String filename = imageFileNames[i];
if (filename.indexOf("Store") == -1) {
PImage temp = loadImage("data/images/"+filename);
images.add(temp);
}
}
println(images.size() + " images loaded!");
}
Get sketch-name
final String sketchName = getClass().getName();
Looping through the pixels of an image
float ratio = float(POSTER_H)/float(POSTER_W);
float tilesX = 90;
float tilesY = ratio * tilesX;
float tileSize = POSTER_W / tilesX;
for (int y = 0; y < comp.height; y += tileSize) {
for (int x = 0; x < comp.width; x += tileSize) {
color c = comp.get(x, y);
float b = map(brightness(c), 0, 255, 1, 0);
}
}
Exporting multiple Videos at once
import com.hamoid.*;
VideoExport videoExport;
VideoExport videoExportCropped;
void setup(){
videoExport = new VideoExport(this, sketchName+".mp4");
videoExport.setFrameRate(30);
videoExport.startMovie();
videoExportCropped = new VideoExport(this, sketchName+"cropped.mp4", p);
videoExportCropped.setFrameRate(30);
videoExportCropped.startMovie();
}
void draw(){
if (frameCount > 1) {
videoExport.saveFrame();
videoExportCropped.saveFrame();
}
}
Related
Please note that this article is still in development Please note: This article may be very time-bound and the items […]

After a long period of work, about a dozen discarded lessons, and many discussions with a wide variety of people, […]
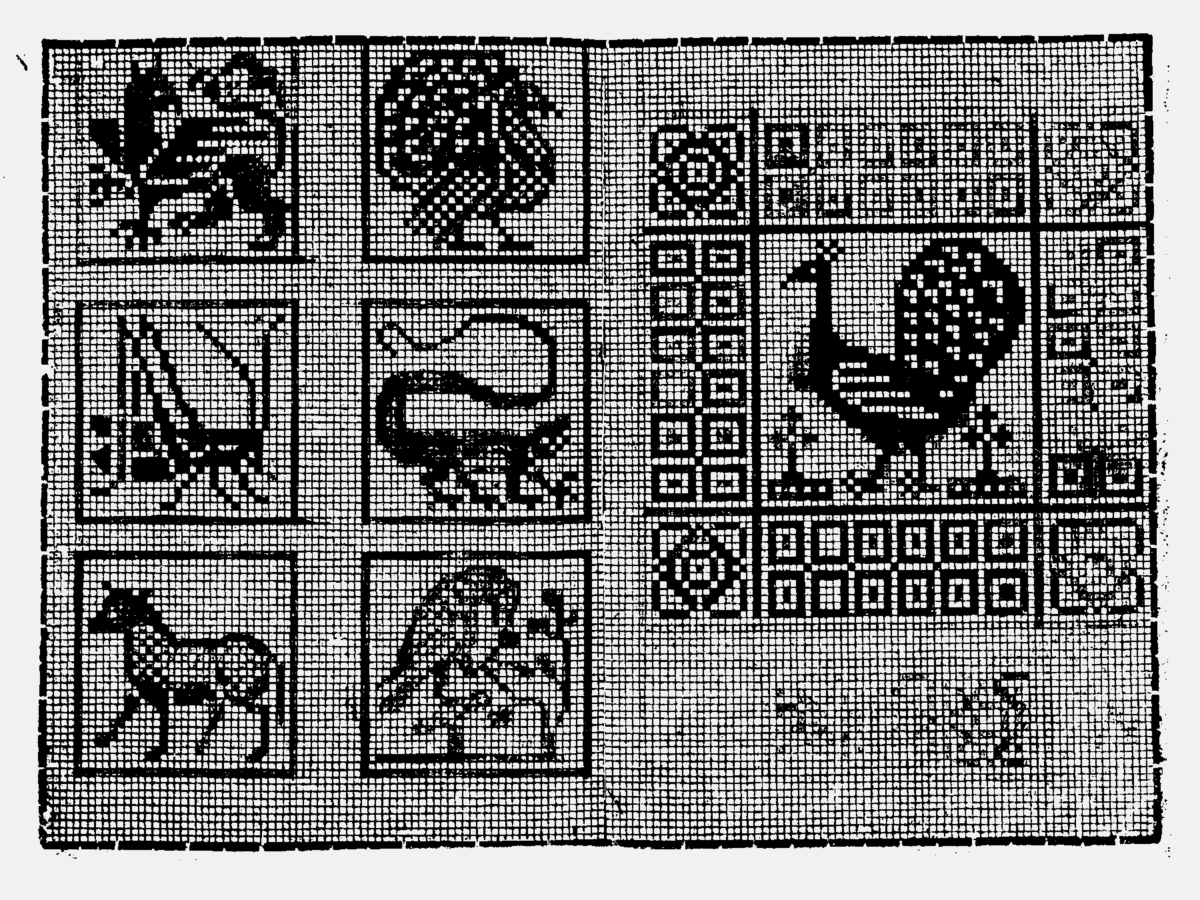
How do you develop a course for a subject as abstract and multifaceted as the topic of grid systems? Either […]
[INTROTEXT VON TIM HIER EINFÜGEN] What sparked your interest in creative coding and how did you get started? For me, […]
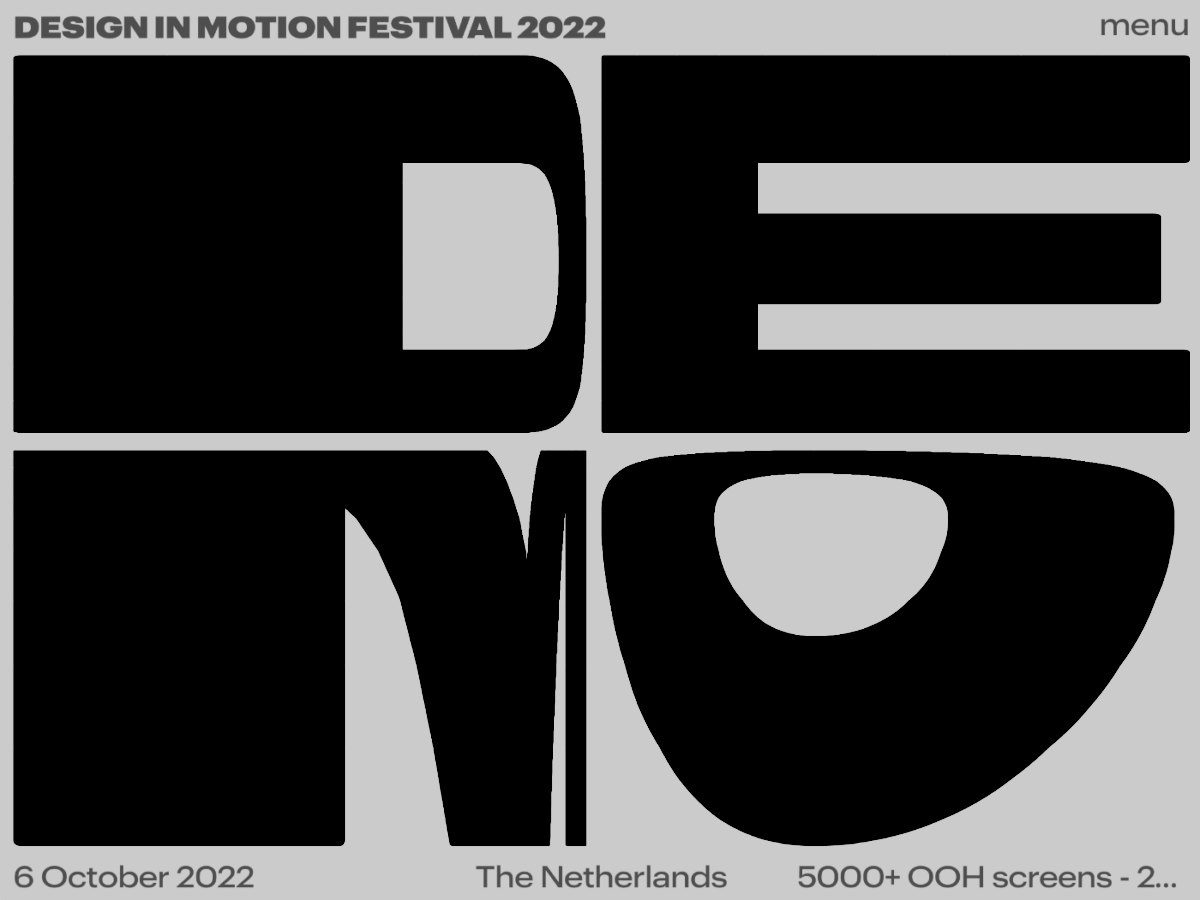
Imagine if the majority of all outdoor displays in public spaces were broadcasting the best of design and moving image […]
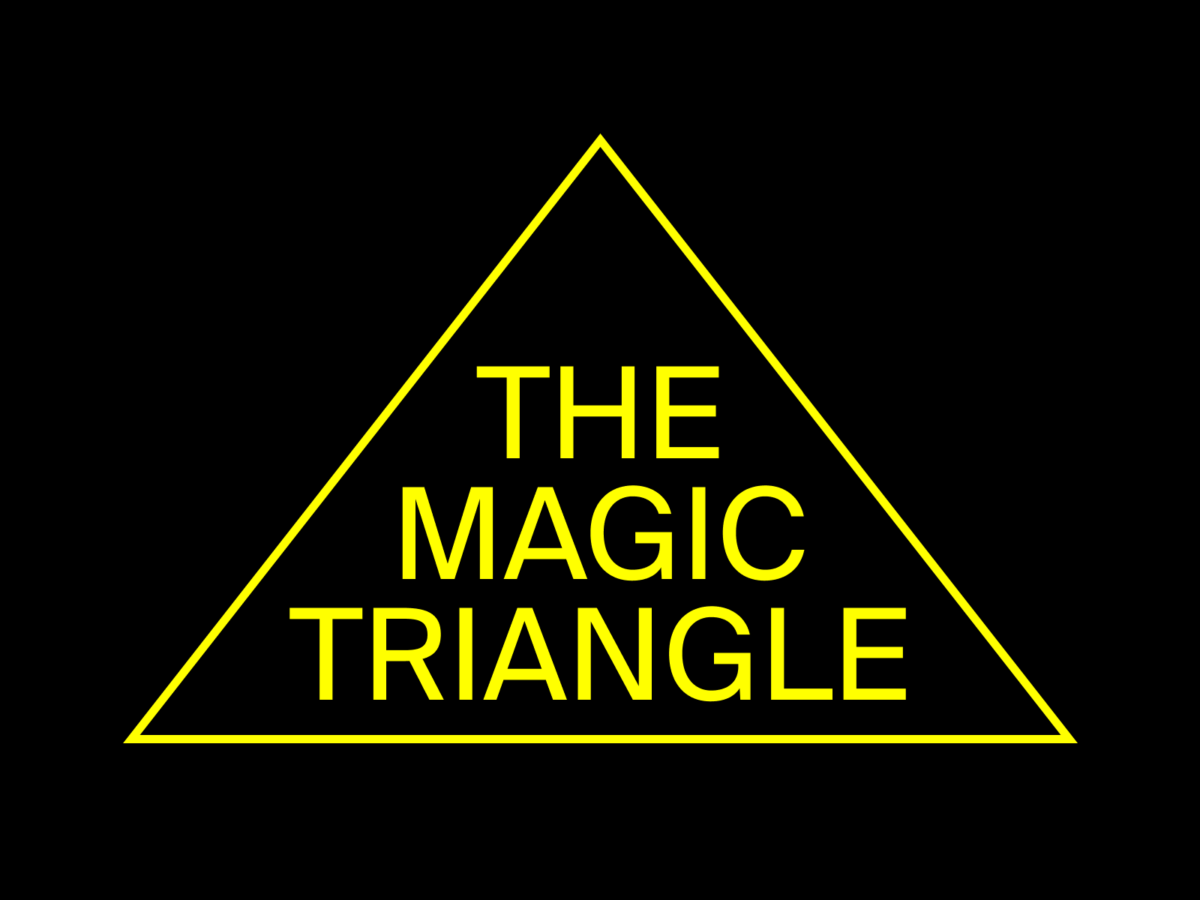
Hey people, I hope you are all well! I escaped the cold and wet weather in Germany and am now […]
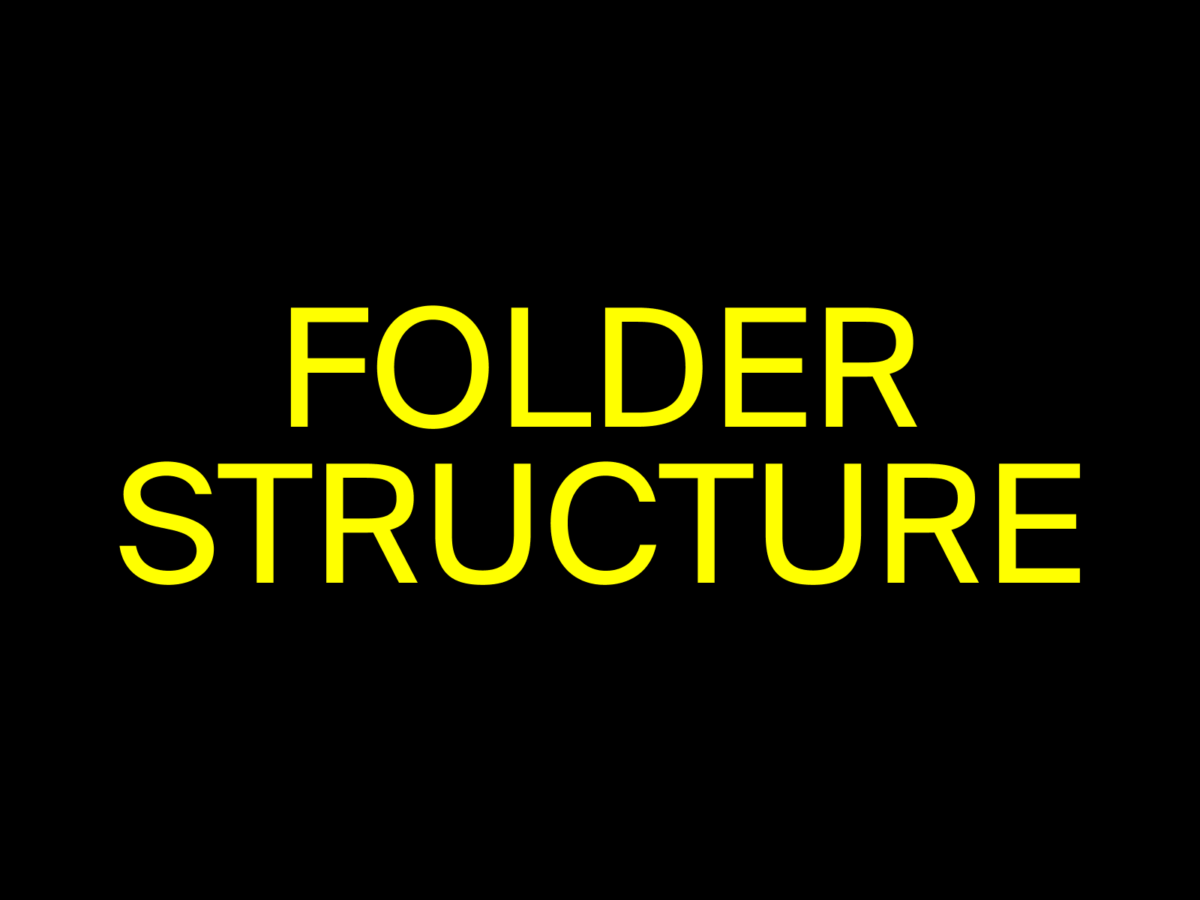
Hey everyone, I hope you are well! Today I would like to talk about a problem in the sketching process. […]