Preview: Random Compositions
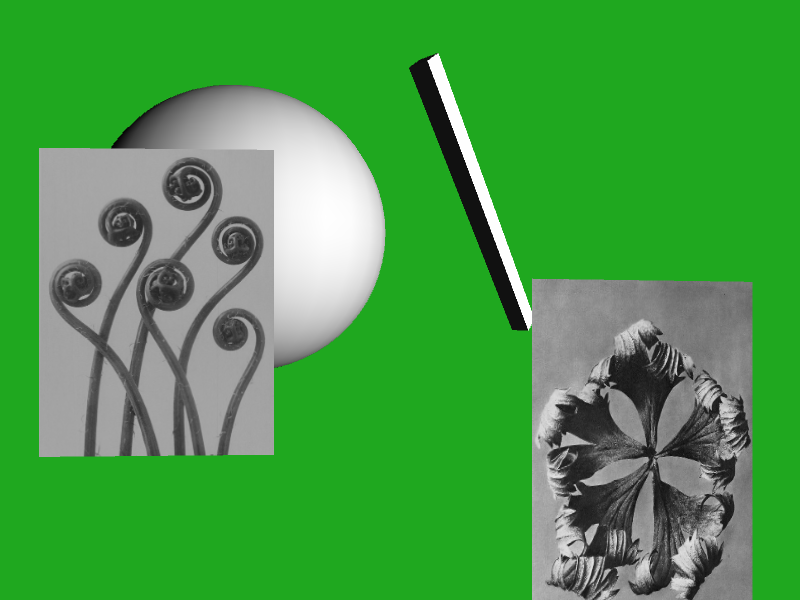
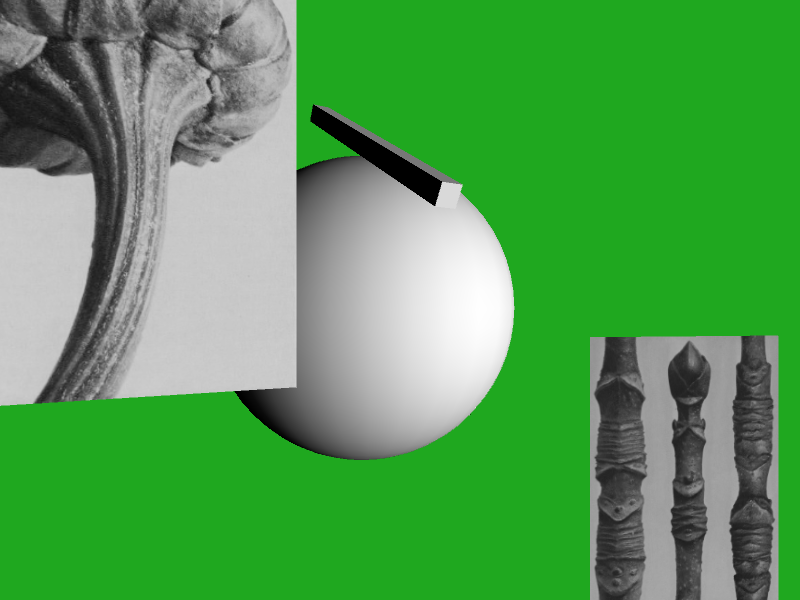

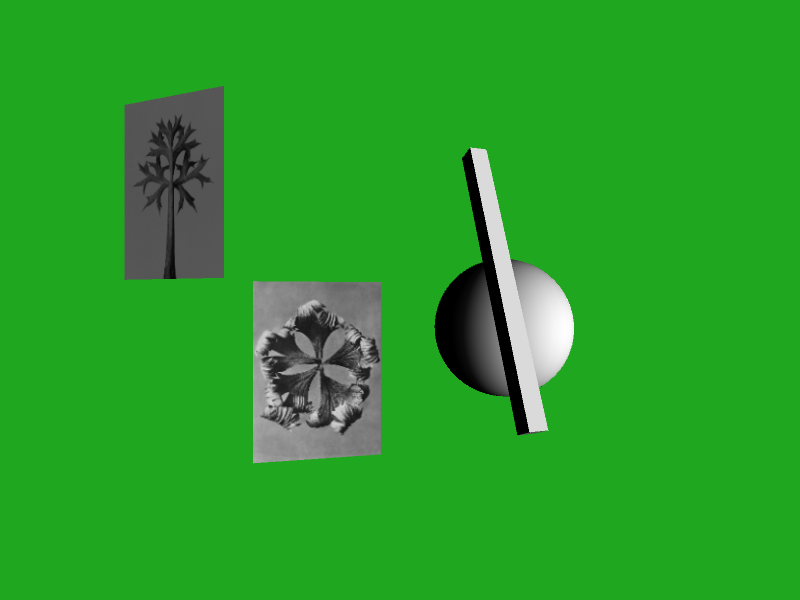
One of the most exciting and maybe even unsettling discoveries in the learning process of Creative Coding in Graphic Design is randomness. It allows you to let the computer make its own, unpredictable decisions. Here two worlds collide, because from a traditional design perspective we are used to have almost full control over the things we create. Randomness can make us feel a bit unsettled and uncertain, but at the same time it reveals the full potencial of coded design: to generate any number of variations within a defined set of constraints. That’s really exciting. In this article I’ll try to show you why.
Random Compositions is how I call an excercise I give quite often to my students. The brief is fairly simple. I ask them to develop an application in which they define three different geometric shapes that get placed randomly on the sketch window. Utilizing the terminology of “Flexible Visual Systems”, a theory and method by my friend Martin Lorenz, these geometric shapes can be called components, whereas the application itself can be called the system.
The most simple solution
The most simple geometric shapes you can create with just a line of code in Processing and p5.js are lines, circles, ellipses, rectangles and squares. While designing the following flexible visual system, I came up with three different geometric shapes as components: A thin line, a circle and a thick line, on which we also could look at as a rectangle.
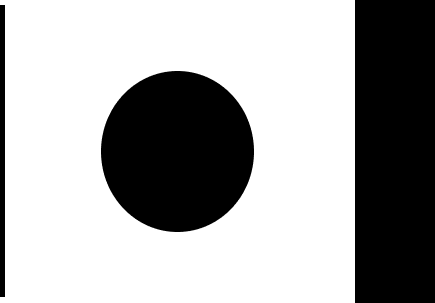
In the following sketch all of the mentioned shapes get positioned randomly on the sketch window. I also used randomness to vary the size of the circles.
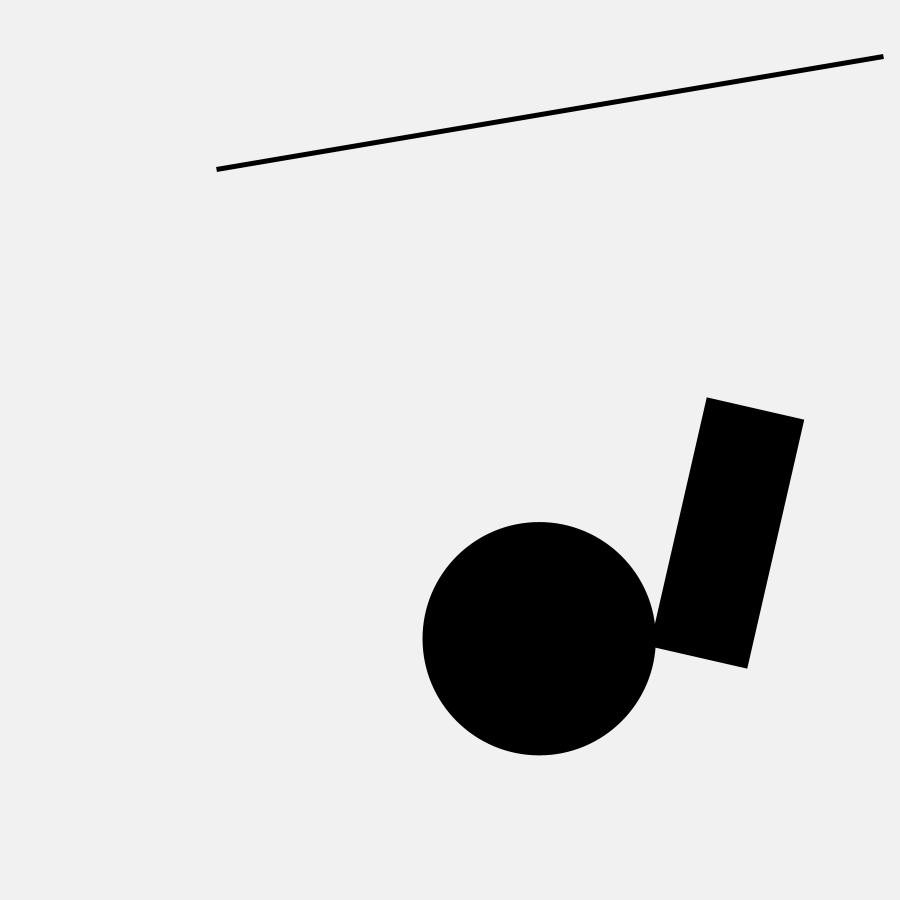
color bg = #f1f1f1;
color fg = #000000;
void setup() {
size(900, 900);
strokeCap(RECT);
}
void draw() {
background(bg);
// line
stroke(fg);
strokeWeight(5);
float lineX1 = random(0, width);
float lineY1 = random(0, height);
float lineX2 = random(0, width);
float lineY2 = random(0, height);
line(lineX1, lineY1, lineX2, lineY2);
// circle
noStroke();
fill(fg);
float circleX = random(0, width);
float circleY = random(0, height);
float diameter = random(200, 300);
circle(circleX, circleY, diameter);
// rectangle
// (I draw a line here but assign a thick stroke weight here)
stroke(fg);
strokeWeight(100);
float line2X1 = random(0, width);
float line2Y1 = random(0, height);
float line2X2 = random(0, width);
float line2Y2 = random(0, height);
line(line2X1, line2Y1, line2X2, line2Y2);
}
This is fascinating, right? Withing seconds, the application can generate hundreds of compositions, each one is a different permutation of exactly the same set of rules, the same system. Next, let’s polish the system a bit, add some padding and apply color.
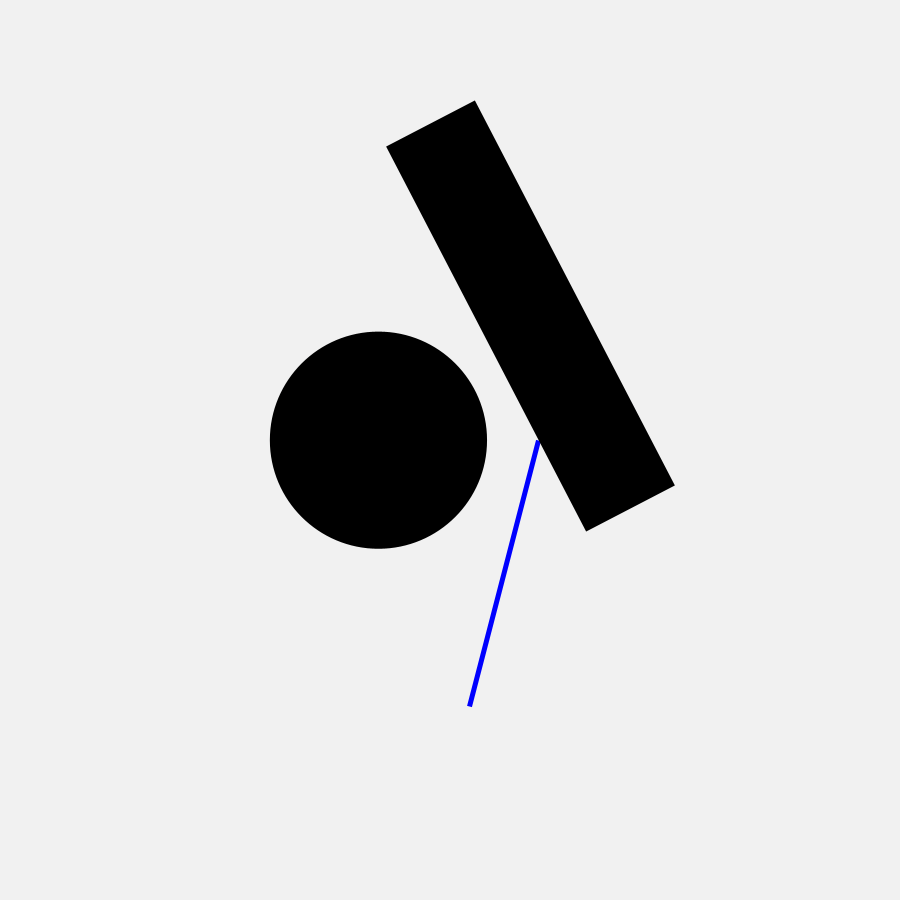
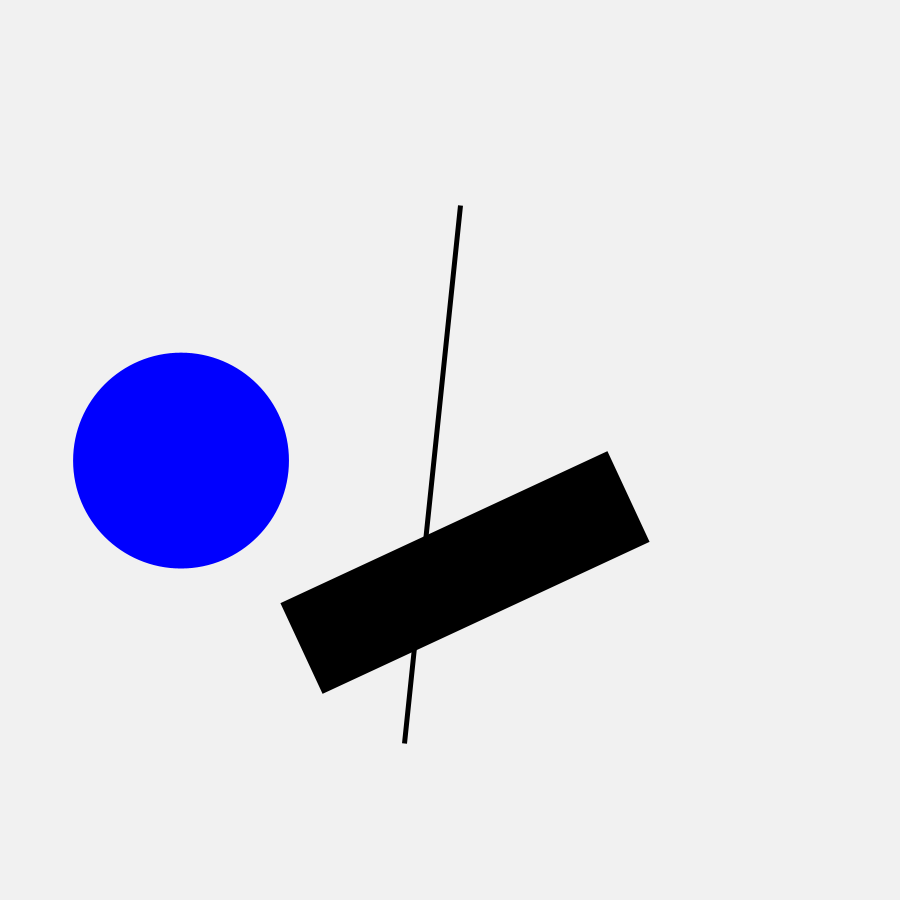
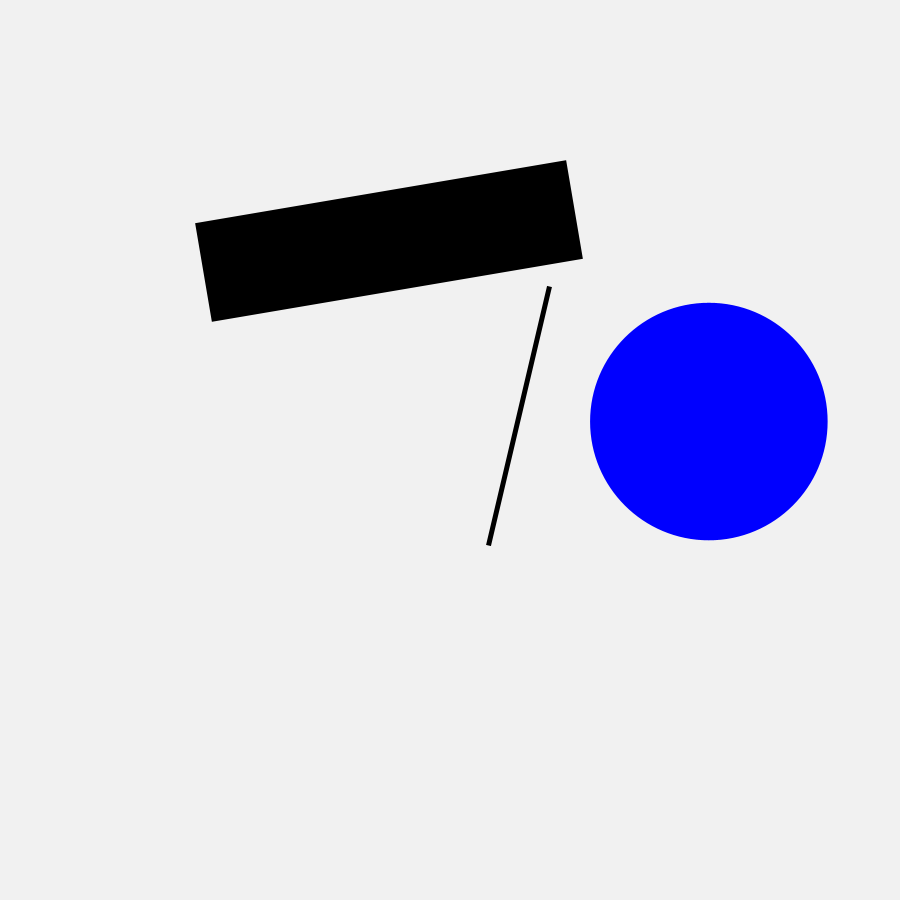
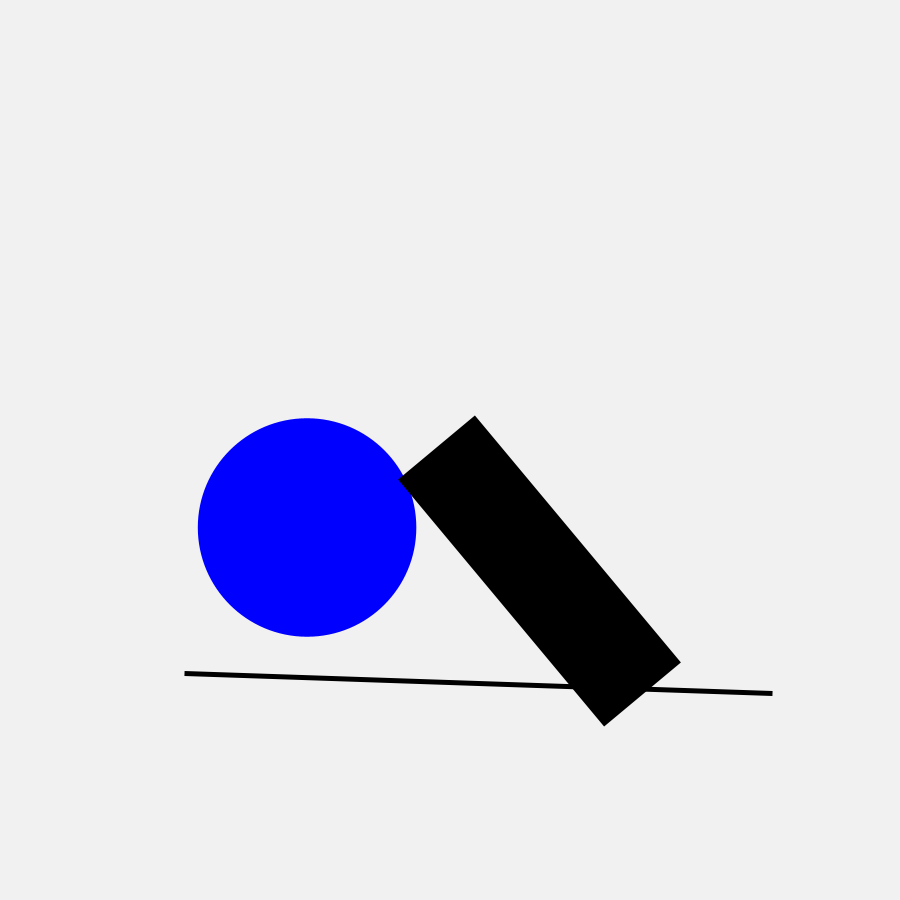
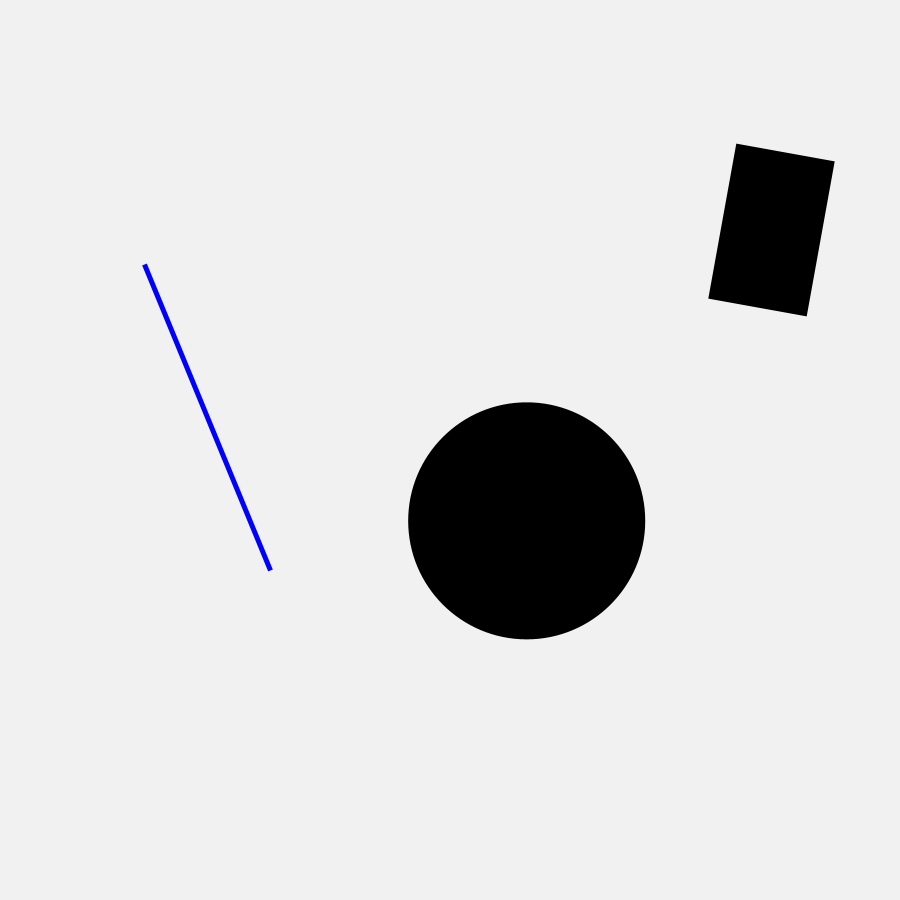
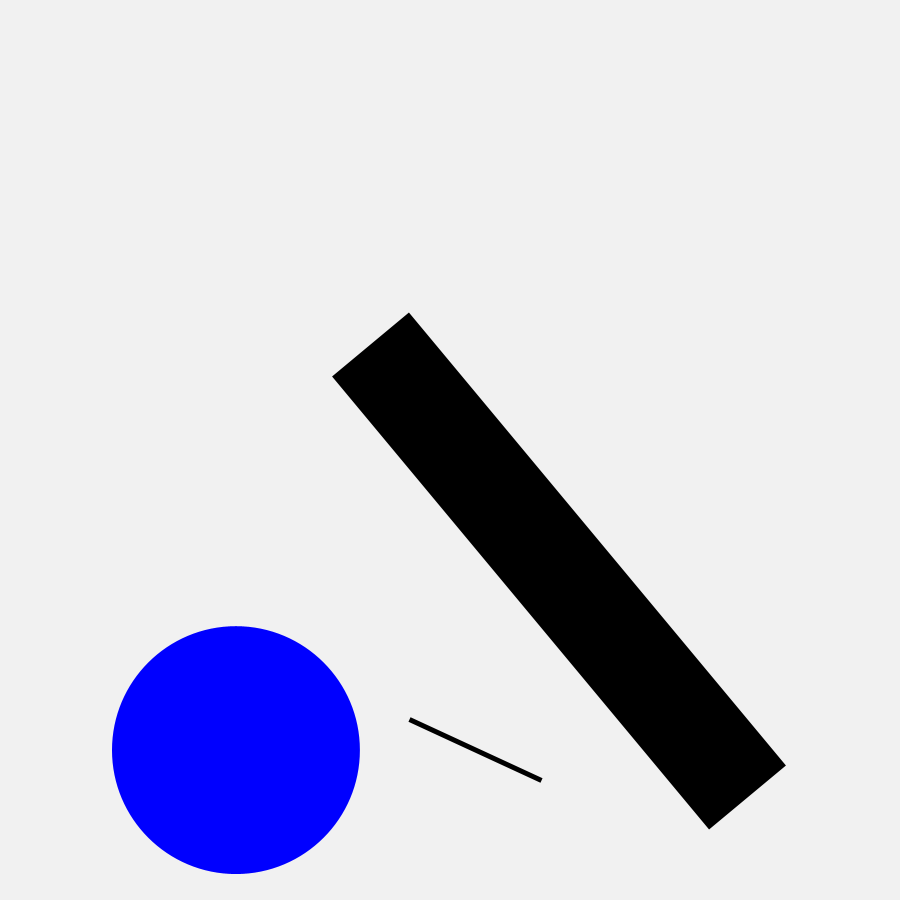
The simple random compositions can already can express some vague ideas. What can you see in these compositions? I see a skier (bottom- eft) a base jumper (top right) and a music conductor (bottom center). Interestingly all the associations are connected to the idea of a human. The compositions look like radically absracted icons. This is actually the simplest way of implementing a random composition. Of course you can utilize any kind of visual element that can be displayed with the given technology.
Adding images
To make the output more interesting, you can add add more meaningful components to the system. A set of images for example. I always have my carefully curated selection of public domain images at hand, that I use for all of my experiments. I have made a essay-film on how I search and find imagery on the internet.
Here we have a nice foundation for a form-based flexible visual Identity, as defined by Martin Lorenz. All three components are composed in a way that the content of the image is always recognizable.
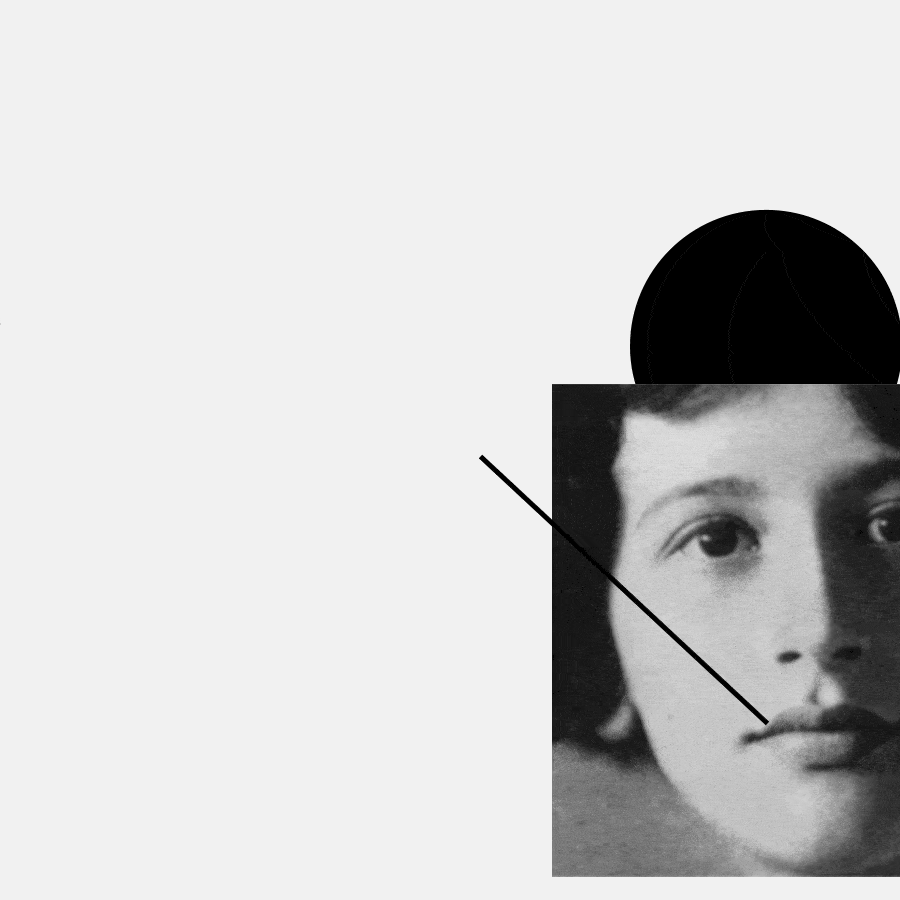
color bg = #f1f1f1;
color fg = #000000;
color accent = #0000ff;
float padding = 100;
void setup() {
size(900, 900);
strokeCap(RECT);
}
void draw() {
int imgSelector = int(random(3));
PImage img = loadImage(imgSelector + ".jpg");
int imageSize = int(random(400, 500));
img.resize(imageSize, 0);
background(bg);
// circle
noStroke();
fill(fg);
float circleX = random(padding, width-padding);
float circleY = random(padding, height-padding);
float diameter = random(200, 600);
circle(circleX, circleY, diameter);
// image
imageMode(CENTER);
push();
translate(random(padding, width-padding), random(padding, height-padding));
image(img, 0, 0);
pop();
// line
stroke(fg);
strokeWeight(5);
float lineX1 = random(padding, width-padding);
float lineY1 = random(padding, height-padding);
float lineX2 = random(padding, width-padding);
float lineY2 = random(padding, height-padding);
line(lineX1, lineY1, lineX2, lineY2);
}
From 2D to 3D
While creating the sketches for this text, many new ideas pop up in my mind. One possibility is to transform the system from 2D into 3D. To do this, I have replaced the circle with a sphere and the line with a box. I also rotated the images slightly at random and let directional light shine into the scene from the right-hand side. The images I used are available on The Public Domain Review.

color bg = #1fa81f;
color fg = #000000;
float padding = 100;
void setup() {
size(586, 810, P3D);
strokeCap(RECT);
}
void draw() {
background(bg);
// circle
noStroke();
fill(#ffffff);
directionalLight(255,255,255,-1,0,-1);
float circleX = random(padding, width-padding);
float circleY = random(padding, height-padding);
float diameter = random(100, 300);
push();
translate(circleX,circleY,-300);
sphere(diameter);
pop();
for (int i = 0; i < 2; i++) {
int imgSelector = int(random(20));
PImage img = loadImage(imgSelector + ".jpg");
int imageSize = int(random(100, 400));
img.resize(imageSize, 0);
// image
imageMode(CENTER);
push();
translate(random(padding, width-padding), random(padding, height-padding));
rotateY(radians(random(-22,22)));
image(img, 0, 0);
pop();
}
// line
float lineX1 = random(padding, width-padding);
float lineY1 = random(padding, height-padding);
push();
translate(lineX1,lineY1);
rotateY(radians(random(360)));
rotateZ(radians(random(360)));
box(300,20,20);
pop();
}
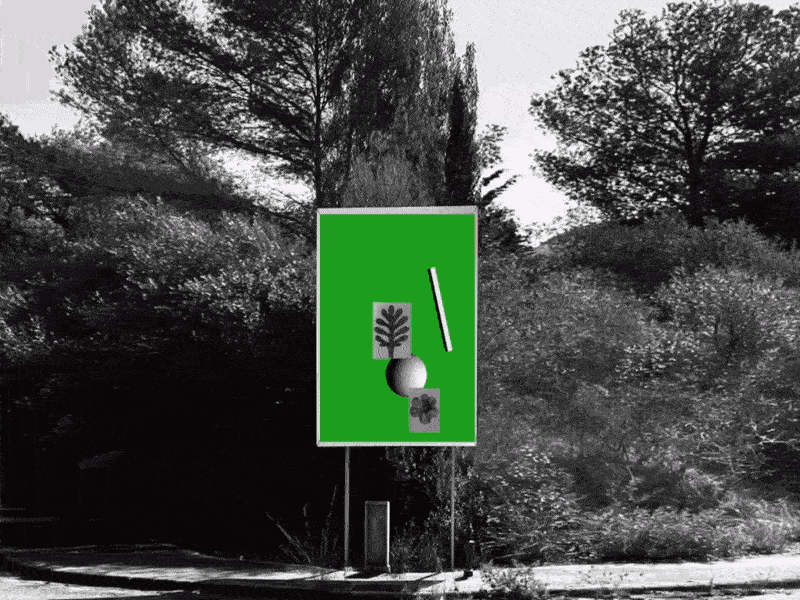
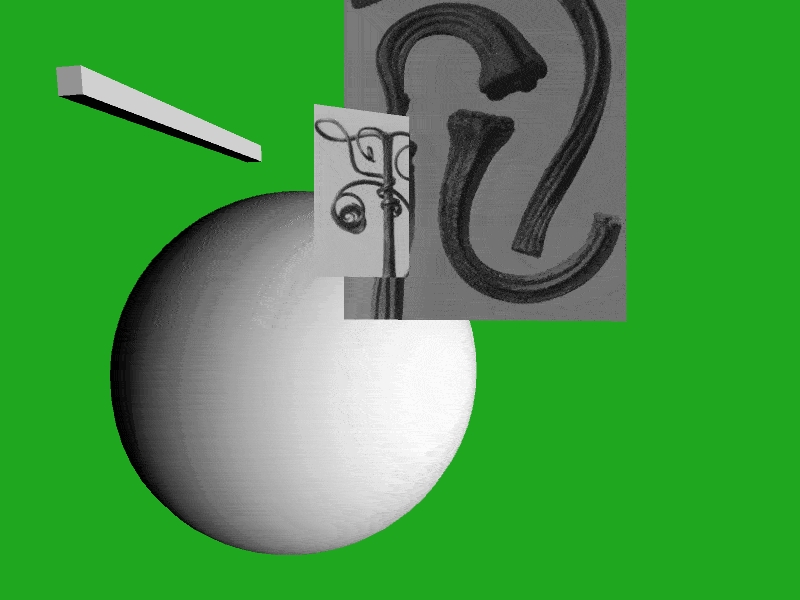
Wrapping up
This is just one possible way to go with the “Random Compositions” challenge. My students surprise me again and again with how they deal with this simple assignment. If this tutorial has given you the impetus to experiment yourself, I would be delighted if you would share the results with me. Simply send an email to feedback@timrodenbroeker.de or post your project on social media with a link to my profile.
Related
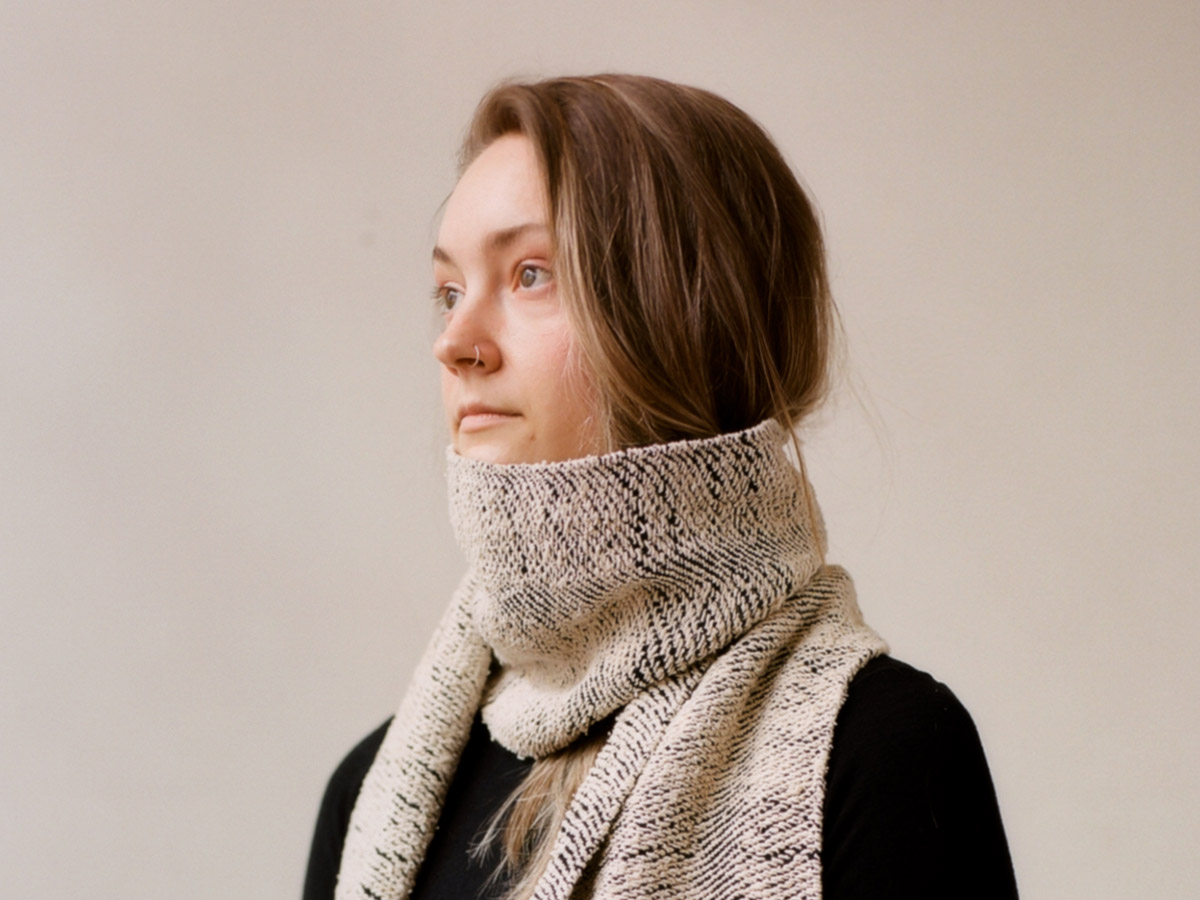
In this post I’d like to introduce you to Sam Griffith, a talented graphic designer based in Detroit, to discuss […]
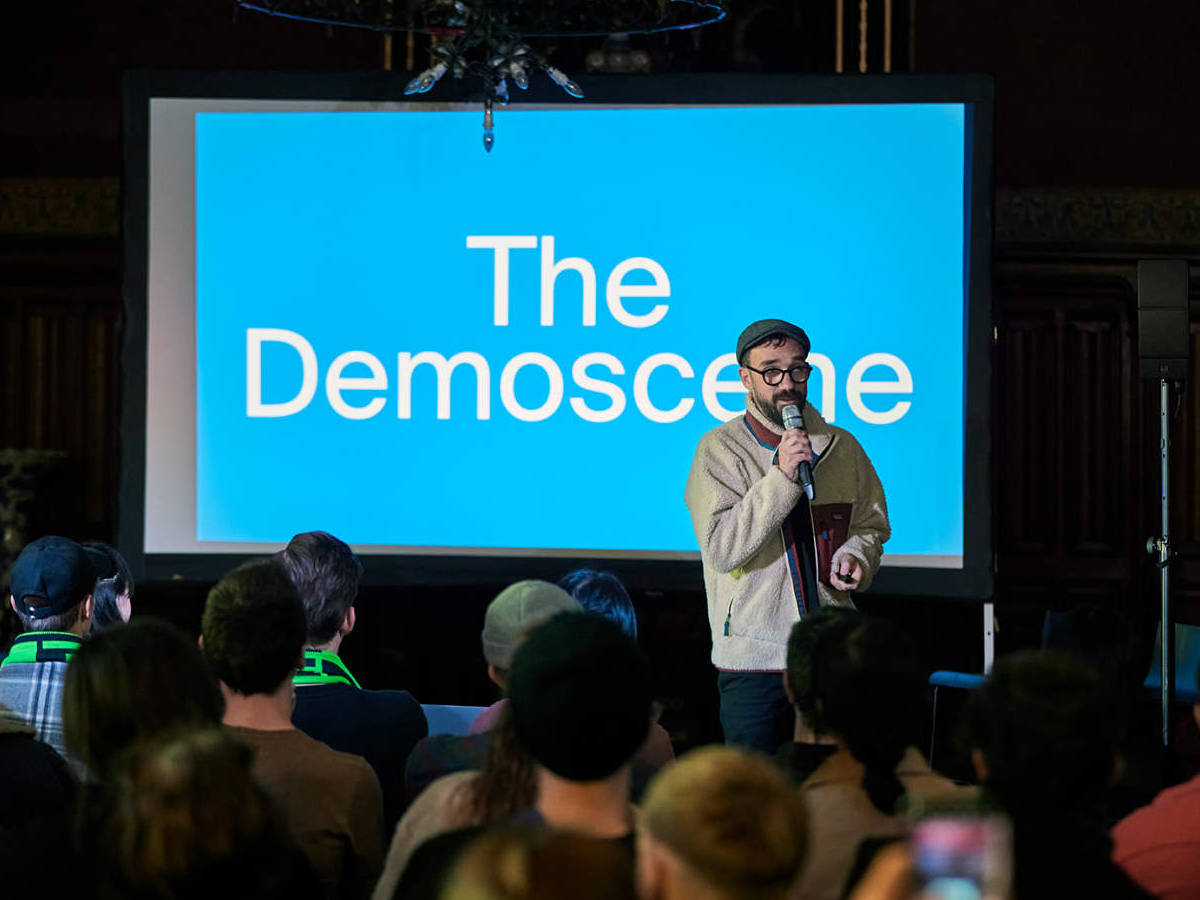
The next edition of the DEMO Festival is already approaching and I am currently developing a brand new talk for […]
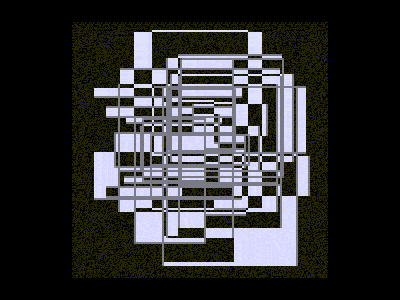
20 = 1 21 = 222 = 323 = 824 = 1625 = 3226 = 6427 = 128 … »In […]
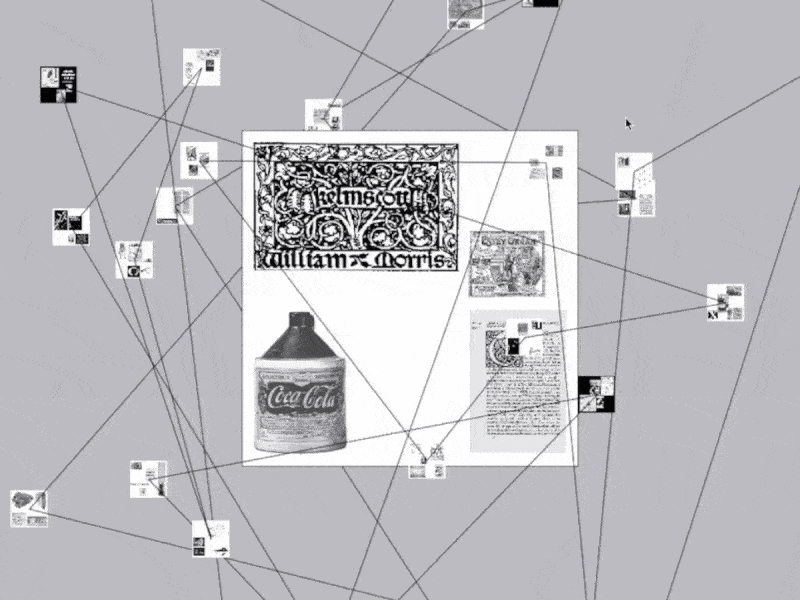
In 2022, I spontaneously posted a story on Instagram: If anyone out there is also in Rotterdam, I’d love to […]
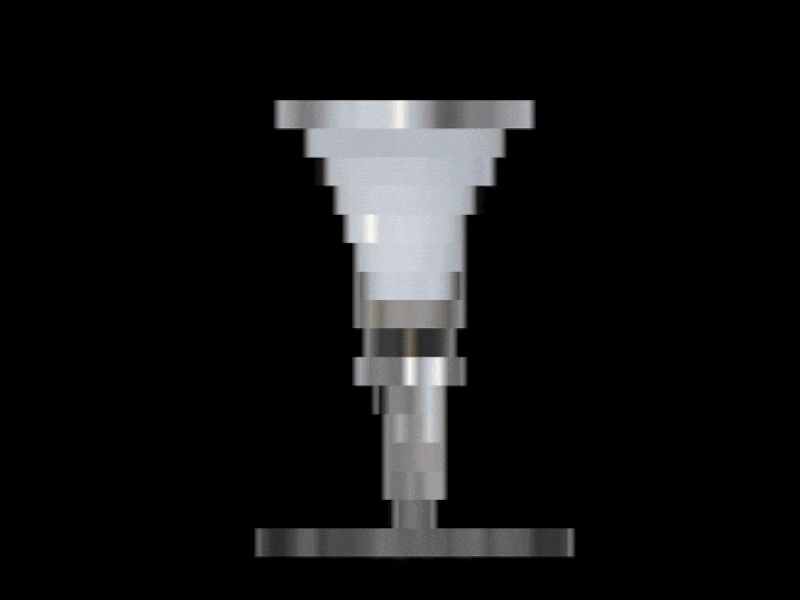
Lena: This 10-minute visualiser for A. G. Cooks album teaser featuring my python archive generator, is one of my favourite […]

One of the first exercises I assign to my students in my seminars is called “Random Compositions”. Basically, it’s quite […]
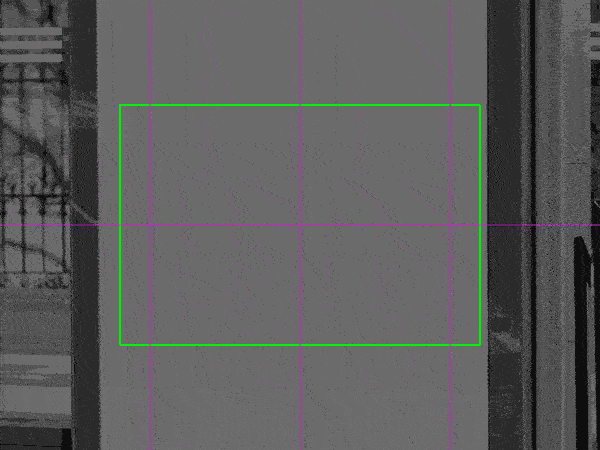
For my students at Elisava, I have created a new version of my mockup-tool. You need two different files for […]
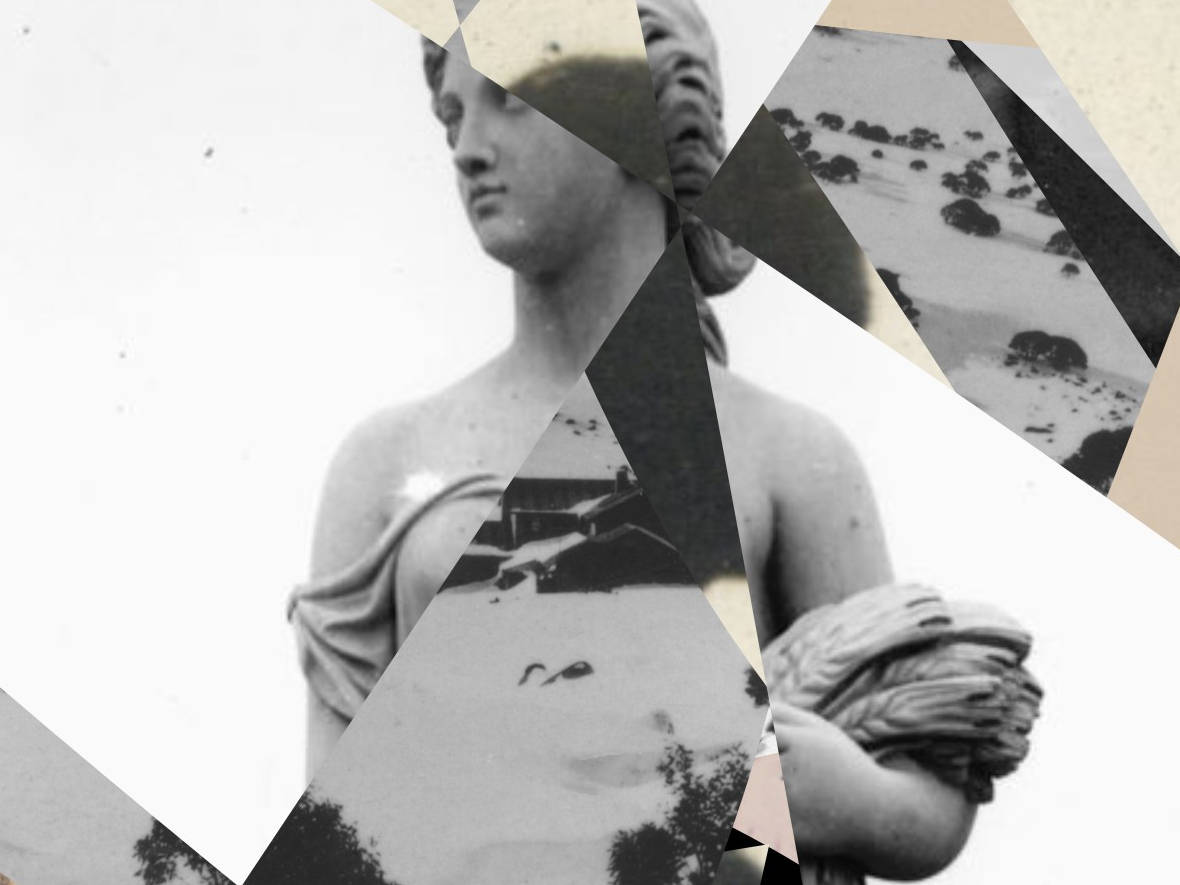
2023-12-01 Today I want to share with you a first prototype that will be the basis for a new course […]